今回はandroidのUIを作成する上で特に使う回数の多い、ボタンについて解説したいと思います。
アンドロイドには様々なボタンがありますが、今回は合計8個のボタンについてその特徴と簡単な使用方法(Kotlin)についてまとめます。
目次
Andorid ボタン一覧(よく使うボタンのみ紹介)
Android studioでは下記8つのボタンがよく使用されます、それぞれのボタンに関して、ボタンの名称、ボタン形状、ボタンの使用場面について簡単にまとめました。
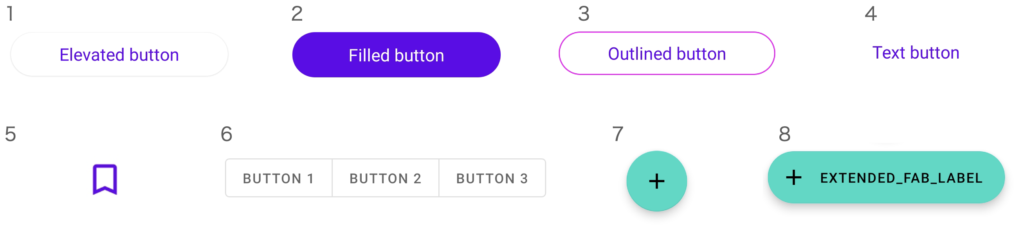
No | ボタン名称 | ボタンの形状 | ボタンの使い方 |
---|---|---|---|
1 | Elevated button | ![]() | 返信、全て表示、カートに追加、ゴミに捨てる |
2 | Filled button | ![]() | 保存、確認、実行 |
3 | Outlined button | ![]() | 返信、全て表示、カートに追加、ゴミに捨てる |
4 | Text button | ![]() | もっと詳しく、全て表示 |
5 | Icon button | ![]() | お気に入りに追加、プリント |
6 | Segmented button | ![]() | 複数の選択肢の中からの選択 |
7 | Floating action button(FAB) | ![]() | 新規追加、作成 |
8 | Extended FAB | ![]() | 新規追加、作成 |
それぞれのボタンの特徴
ここからは今あげた8つのボタンの特徴と簡単な使用方法をそれぞれ紹介していきたいと思います。
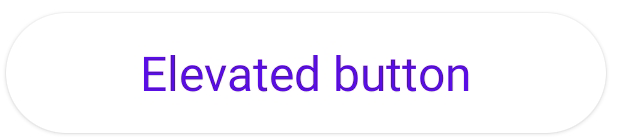
Elevated button の特徴
Elevated button は基本的にはボタンの背後にシャドウを持つ塗りつぶされたボタンです。
影がついているため他のボタンと比べて存在感を持っており、デザインの中でも比較的目立つボタンです。
Elevated button のコード
<Button
android:id="@+id/elevatedButton"
style="@style/Widget.Material3.Button.ElevatedButton"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="Elevated button"
android:textSize="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
Elevated buttonのさらに詳しい使い方についてはこちらにまとめています。
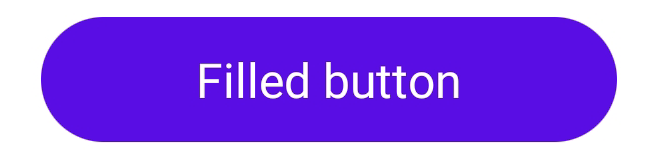
Filled button の特徴
Filled button はボタンの背景が基本的に単色で塗られている一般的なボタン。Android studioのパレットからボタンを追加するとこの形のボタンが作成されます。
Filled button は Floating action button(FAB) の次に視覚的なインパクトがあるボタンとなっており、「保存」「今すぐ参加」「確認」などのフローを完結させる時などの重要な最終アクションの際に使用するボタンです。
Filled button のコード
<Button
style="@style/Widget.Material3.Button.IconButton.Filled"
android:id="@+id/filledButton"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="Filled button"
android:textSize="16dp"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
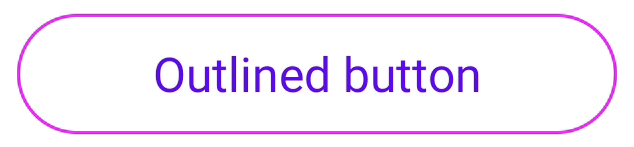
Outlined button の特徴
Outlined button はボタンの外周にラインが引いてあり、ボタンの中は塗りつぶされていないです。
Filled button よりも目立つボタンではないため、アプリの主要ではないアクションで使用されるボタンになります。
Filled button と組み合わせて使用することでFilled button の代替となる、2次的なアクションの選択肢として使用されることが多いです。
Outlined button のコード
<Button
style="@style/Widget.Material3.Button.OutlinedButton"
android:id="@+id/outlinedButton"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="Outlined button"
android:textSize="16dp"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
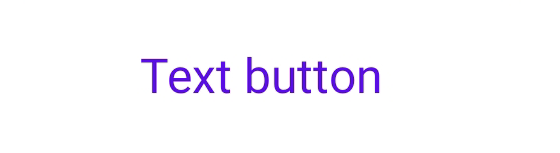
Text button の特徴
Text button は複数の選択肢を提示する場合などの最も優先度の低いアクションに使用されることが多いです。また、塗りつぶしが何もないボタンのため様々な背景に設置することが可能です。
Text button はカード、ダイヤログ、スナックバーなどのコンポーネントに埋め込まれて使用されることが多いです。Text buttonの色とスタイルはボタン以外のテキストや要素から常に認識できるようなデザインにする必要があります。
Text button のコード
<Button
style="@style/Widget.Material3.Button.TextButton"
android:id="@+id/textButton"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="Text button"
android:textSize="16dp"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
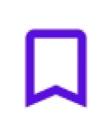
Icon button の特徴
Icon button はコンパクトなレイアウトでアクションを表示する時に使用します。
アイコンボタンはオーバーフローメニューや検索などのオープニングアクション、お気に入りやブックマークなどのトグルでオン/オフができるバイナリアクションを表現できる。
Icon button のコード
app:iconでボタンに使用するアイコンを設定します。そのためresのdrawableフォルダに使用したいアイコンを入れておきましょう。
<Button
style="@style/Widget.Material3.Button.IconButton"
android:id="@+id/iconButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:icon="@drawable/ic_baseline_bookmark_border_24"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent" />

Segmented button の特徴
Segmented button オプションの選択、ビューの切り替え、要素の並べ替えなどのアクションで使用されるボタンです。
Segmented button のコード
<com.google.android.material.button.MaterialButtonToggleGroup
android:id="@+id/toggleButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
>
<Button
style="?attr/materialButtonOutlinedStyle"
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 1"
/>
<Button
style="?attr/materialButtonOutlinedStyle"
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 2"
/>
<Button
style="?attr/materialButtonOutlinedStyle"
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 3"
/>
</com.google.android.material.button.MaterialButtonToggleGroup>
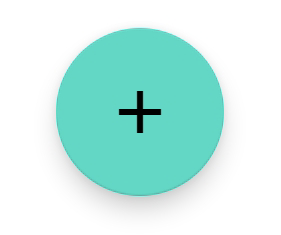
Floating action button の特徴
Floating action button は名称が長いためFABという短縮名で呼ばれることが多いです。
FABは画面上で最も重要なアクションで使用されます。そのため、FABは画面上の全てのコンテンツの前に表示され丸みを帯びた形状とアイコンによって表示されます。
Floating action button のコード
app:srcCompatでボタンに使用するアイコンを設定します。そのためresのdrawableフォルダに使用したいアイコンを入れておきましょう。
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/floating_action_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="16dp"
android:contentDescription="fab_content_desc"
app:srcCompat="@drawable/ic_baseline_add_24"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>
Extended FAB
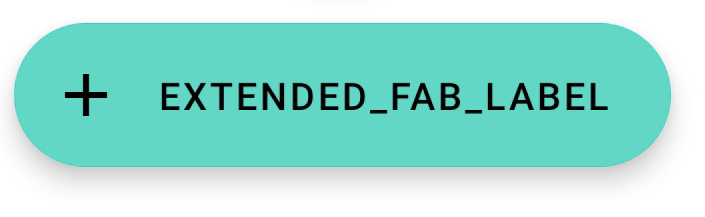
Extended FAB の特徴
Extended FAB はFAB(Floating action button)よりも幅が広くテキストラベルがあるため、FABよりも視覚的に目立つボタンになっています。FABと同様に画面上の全てのコンテンツの前に表示され丸みを帯びた形状とアイコン、テキストによって表示されます。FABでは小さすぎるような画面で使用されることが多いです。
Extended FAB のコード
app:srcCompatでボタンに使用するアイコンを設定します。そのためresのdrawableフォルダに使用したいアイコンを入れておきましょう。
<com.google.android.material.floatingactionbutton.ExtendedFloatingActionButton
android:id="@+id/extended_fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:layout_gravity="bottom|end"
android:contentDescription="extended_fab_content_desc"
android:text="extended_fab_label"
app:icon="@drawable/ic_baseline_add_24"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>